安裝 spatie/image
composer require spatie/image
安裝 spatie/laravel-image-optimizer
composer require spatie/laravel-image-optimizer
php artisan vendor:publish --provider="Spatie\LaravelImageOptimizer\ImageOptimizerServiceProvider"
使用優化功能必須在伺服器上安裝相關工具才能讓圖檔優化,參考說明安裝。
圖檔縮小操作及優化
use Spatie\Image\Image;
//若圖檔寬度大於1280則將圖檔按照原始比例寬度降低到1280
//若圖檔高度大於1024則將圖檔按照原始比例高度降低到1024
//也就是說先依照寬度作降低,若高度還高於1024時,則在依高度降低
Image::load('test.jpg')
->width(1280)
->height(1024)
->save('test.jpg');
//優化圖檔
ImageOptimizer::optimize('test.jpg');
圖檔優化設定
<?php
use Spatie\ImageOptimizer\Optimizers\Cwebp;
use Spatie\ImageOptimizer\Optimizers\Gifsicle;
use Spatie\ImageOptimizer\Optimizers\Jpegoptim;
use Spatie\ImageOptimizer\Optimizers\Optipng;
use Spatie\ImageOptimizer\Optimizers\Pngquant;
use Spatie\ImageOptimizer\Optimizers\Svgo;
return [
/*
* When calling `optimize` the package will automatically determine which optimizers
* should run for the given image.
*/
'optimizers' => [
Jpegoptim::class => [
'-m85', // set maximum quality to 85%
'--strip-all', // this strips out all text information such as comments and EXIF data
'--all-progressive', // this will make sure the resulting image is a progressive one
],
Pngquant::class => [
'--force', // required parameter for this package
'-q 85', //quality factor that brings the least noticeable changes.
],
Optipng::class => [
'-i0', // this will result in a non-interlaced, progressive scanned image
'-o2', // this set the optimization level to two (multiple IDAT compression trials)
'-quiet', // required parameter for this package
],
Svgo::class => [
'--disable=cleanupIDs', // disabling because it is know to cause troubles
],
Gifsicle::class => [
'-b', // required parameter for this package
'-O3', // this produces the slowest but best results
],
Cwebp::class => [
'-m 6', // for the slowest compression method in order to get the best compression.
'-pass 10', // for maximizing the amount of analysis pass.
'-mt', // multithreading for some speed improvements.
'-q 90', // quality factor that brings the least noticeable changes.
],
],
/*
* The directory where your binaries are stored.
* Only use this when you binaries are not accessible in the global environment.
*/
'binary_path' => '',
/*
* The maximum time in seconds each optimizer is allowed to run separately.
*/
'timeout' => 60,
/*
* If set to `true` all output of the optimizer binaries will be appended to the default log.
* You can also set this to a class that implements `Psr\Log\LoggerInterface`.
*/
'log_optimizer_activity' => false,
];
測試
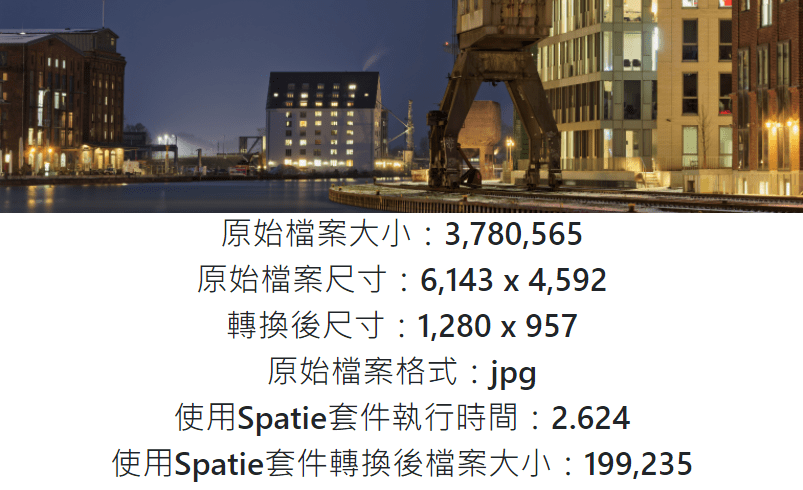
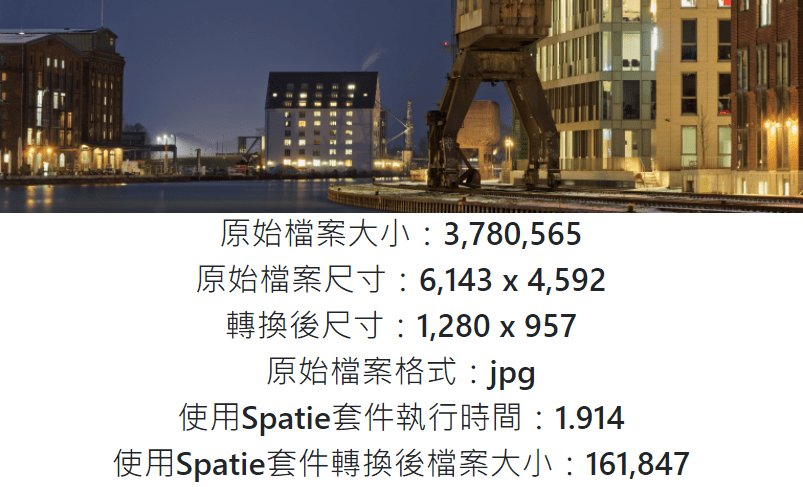
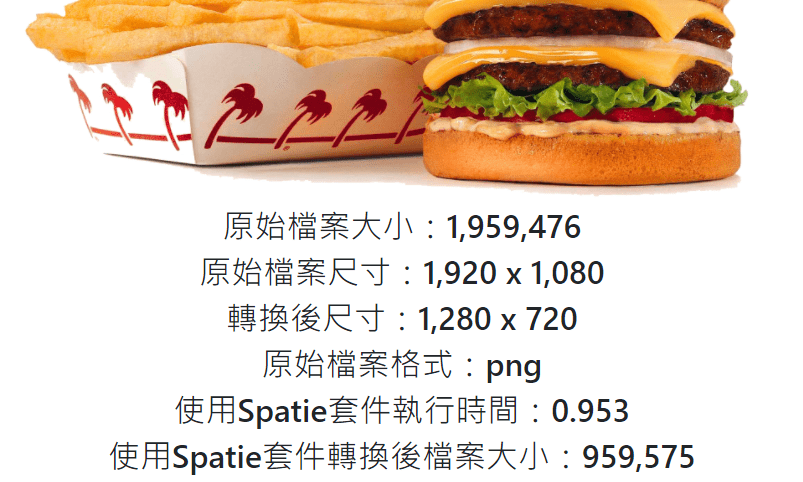
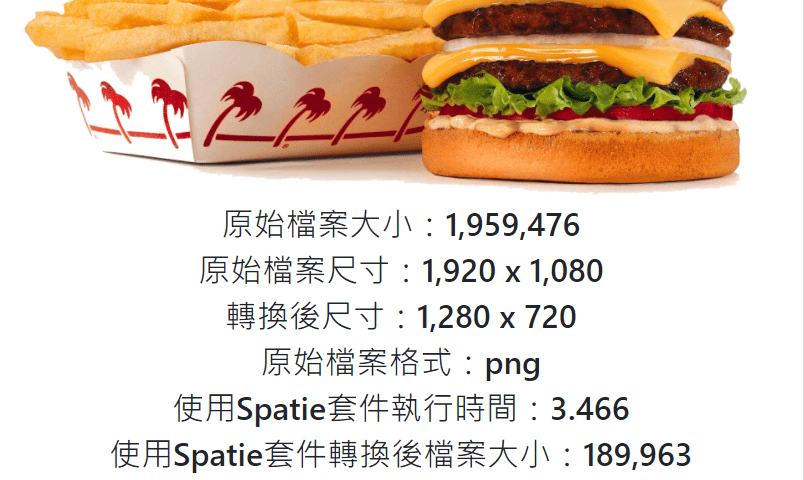
以上可以看出,優化圖檔在 Windows 測試環境中並沒有運作,因為沒有安裝相關的轉換套件。尤其在 PNG 圖檔相差更大。
使用 middleware
此優化套件可以將其註冊在 middleware 中,只要是圖形檔案都可以經過該middleware優化。
// app/Http/Kernel.php
protected $routeMiddleware = [
...
'optimizeImages' => \Spatie\LaravelImageOptimizer\Middlewares\OptimizeImages::class,
];
Route::middleware('optimizeImages')->group(function () {
// all images will be optimized automatically
Route::post('upload-images', 'UploadController@index');
});